Pipeline - 연속된 변환을 일괄적으로 처리하기 위해서 사용된다.
파이프라인을 이용하면 전처리과정과 학습, 예측의 전과정을 통합가능하다.
하지만 뒤에 있을 모델 세부튜닝을 위해 전처리만 적용하려한다.
사용 데이터는 iris 데이터이다.
기본적인 구조는 다음과 같다.
from sklearn.pipeline import Pipeline
new_pipeline = Pipeline([
('Name', tool),('Name', tool)
])
이를 토대로 간단한 파이프라인을 작성하겠다.
sklearn의 SimpleImputer와 StandardScaler를 적용한 예시이다.
from sklearn.preprocessing import StandardScaler
from sklearn.impute import SimpleImputer
new_pipeline = Pipeline([
('imputer', SimpleImputer(strategy = 'mean')),('std_scaler', StandardScaler())
])
결과는 다음과 같다.
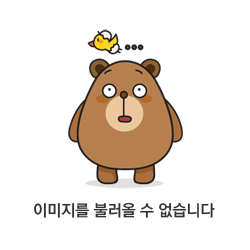
통합적인 변환 이외에도 각각의 열에 대해서 변환을 적용시켜야하는 경우가 생긴다.
이 경우 ColumnTransformer를 이용한다.
- Columntransformer
예시)
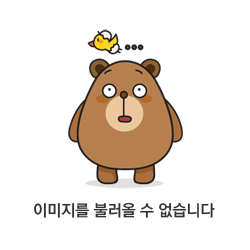
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import OneHotEncoder
num_att = ['col3']
cat_att = ['col1','col2']
col_pipeline = ColumnTransformer([
('num',new_pipeline,num_att),
('cat',OneHotEncoder(),cat_att)
])
df_prepared = col_pipeline.fit_transform(my_df)
결과.
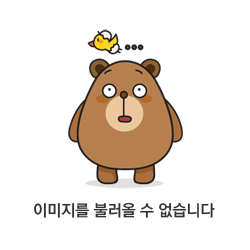
이번 포스팅은 'Hands-On Machine Learning with Scikit-Learn, Keras & TensorFlow 2판' 을 참고하였습니다.
'Others > Data Science' 카테고리의 다른 글
Preprocessing (4) Scaling (0) | 2022.07.19 |
---|---|
Preprocessing (3) Encoding (0) | 2022.07.18 |
Preprocessing (2) 누락된 값의 처리(Null) (0) | 2022.07.15 |
Pandas - DataFrame (3) 조회(loc/iloc) (0) | 2022.07.15 |
Pandas - DataFrame (2) 삭제 (0) | 2022.07.14 |