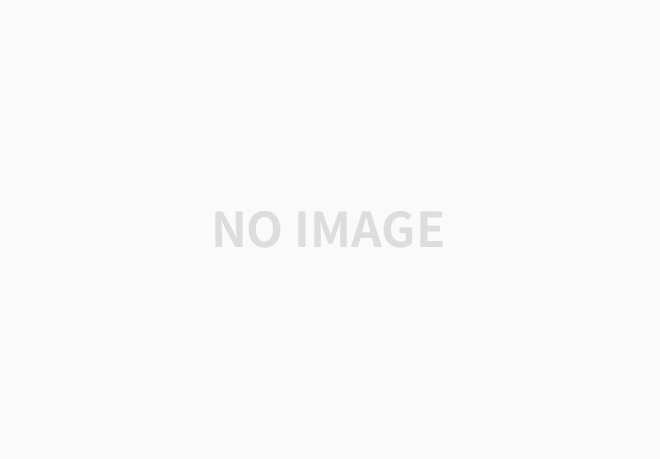
오늘의 학습 키워드: 탐색, BFS
문제: 촌수계산
https://www.acmicpc.net/problem/2644
풀이
촌수의 계산을 위해서 각 노드를 탐색하는 BFS를 이용해 풀이했습니다.
각 노드의 번호와 시작 노드로부터의 떨어진 거리를 담는 큐를 이용했고,
이미 방문한 노드에 재방문하지 않기 위해 visited를 사용했습니다.
import sys
from collections import deque
N = int(sys.stdin.readline().strip())
a, b = map(int,sys.stdin.readline().strip().split())
m = int(sys.stdin.readline().strip())
graph = []
for _ in range(m):
tmp = list(map(int,sys.stdin.readline().strip().split()))
graph.append(tmp)
q = deque([])
q.append([a,0])
tk = 0
visited = []
while q:
m,count = q.popleft()
if m == b:
print(count)
tk = 1
break
else:
for s,e in graph:
if s == m and [s,e] not in visited:
visited.append([s,e])
q.append([e,count+1])
elif e == m and [e,s] not in visited:
visited.append([e,s])
q.append([s,count+1])
if tk == 0:
print(-1)
회고
오랜만에 기본적인 BFS문제를 풀어보았습니다. 문제가 잘 풀려서 다행이라 생각합니다.
내일 학습할 것.
SQL, Python, 독서
'코딩테스트 > 알고리즘' 카테고리의 다른 글
99클럽 코테 스터디 19일차 TIL (구명보트 - 프로그래머스) (0) | 2024.08.09 |
---|---|
99클럽 코테 스터디 18일차 TIL (단지번호붙이기 boj 2667) (0) | 2024.08.08 |
99클럽 코테 스터디 16일차 TIL (모음사전) (0) | 2024.08.06 |
99클럽 코테 스터디 15일차 TIL (745. Prefix and Suffix Search) (0) | 2024.08.06 |
99클럽 코테 스터디 14일차 TIL (숫자 카드2) (0) | 2024.08.04 |